Have you ever felt frustrated by the clunky experience of traditional page reloads in WordPress? Every time a user interacts with a page element, the entire page refreshes, creating a disjointed and sluggish experience. This is where AJAX (Asynchronous JavaScript and XML) comes in as a game-changer. AJAX allows for dynamic content updates in WordPress, meaning specific sections of a page can be refreshed without a full reload, resulting in a smoother and more responsive user experience. Additionally, AJAX can improve website performance by reducing the amount of data transferred between the server and the client.
In this blog post, we’ll delve into the world of AJAX for WordPress. We’ll begin by understanding the core concepts of AJAX and its role in dynamic content updates. Then, we’ll embark on a practical journey, building a simple AJAX implementation that updates a shopping cart total dynamically. Following that, we’ll explore advanced techniques, discuss security considerations, and delve into using AJAX with other WordPress functionalities. Finally, we’ll conclude by summarizing the benefits of AJAX and provide resources for further exploration.
Understanding the AJAX Process
AJAX works by establishing an asynchronous communication channel between a web page and the server. When a user interacts with a page element, typically a button or link, JavaScript code initiates an AJAX request. This request is sent to a specific URL on the server, often containing data relevant to the interaction. The server then processes the request, performs the necessary actions (e.g., database queries, calculations), and returns a response in a format like JSON (JavaScript Object Notation). Finally, the JavaScript code on the client-side (web browser) receives and interprets the response, allowing for dynamic updates to the page’s content without a full reload. This seamless communication between the client and server fosters a more engaging and responsive user experience.
Here in WordPress, we can leverage built-in functions like wp_ajax_url
to retrieve the correct URL for sending AJAX requests. Additionally, functions like wp_send_json_success
simplify the process of returning JSON responses from the server.
Building Your First AJAX Implementation
Let’s solidify our understanding of AJAX by building a practical example. Imagine an e-commerce scenario where you want to update the shopping cart total dynamically whenever a user adds a product to their cart. This eliminates the need for a full page reload, maintaining a smooth user experience and encouraging continued browsing.
First, we need to establish the HTML structure for our shopping cart. This will involve elements displaying product information (e.g., name, price) and a prominent button for adding items to the cart. Additionally, we’ll include a dedicated section showcasing the current shopping cart total.
HTML
<div class="product-info">
<h2>Product Name</h2>
<p>Price: £10.00</p>
<button id="add-to-cart">Add to Cart</button>
</div>
<div id="cart-total">
<h2>Cart Total: £0.00</h2>
</div>
Next, we’ll create a JavaScript function that will handle the AJAX request and response. This function will be triggered when the user clicks the “Add to Cart” button. Here, we can utilize libraries like jQuery for a more concise approach.
JavaScript
$(document).ready(function() {
$("#add-to-cart").click(function() {
// Get the product ID from the product information
var productId = $(this).data("product-id"); // Assuming product ID is stored in a data attribute
// Initiate the AJAX request
$.ajax({
url: ajaxurl, // Using WordPress function to get the correct AJAX URL
type: "post",
data: {
action: "my_custom_action", // Custom action hook to be triggered on the server
product_id: productId
},
success: function(response) {
// Handle the successful response from the server
updateCartTotal(response.data.total); // Assuming response contains updated total in data.total
},
error: function(jqXHR, textStatus, errorThrown) {
// Handle any errors during the AJAX request
console.error("AJAX Error: " + textStatus + " - " + errorThrown);
}
});
});
});
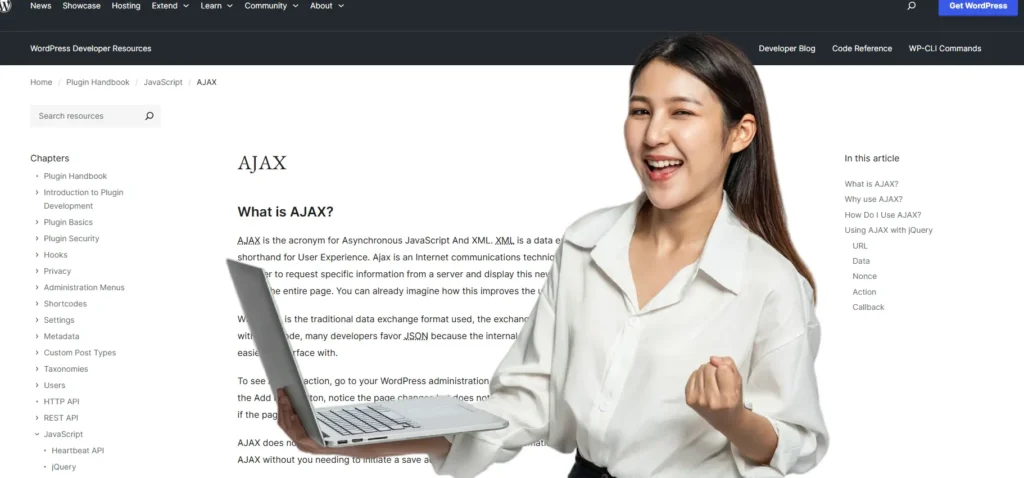
Now, let’s shift our focus to the server-side processing. We’ll achieve this by creating a custom action hook in WordPress using PHP. This hook will be triggered when the AJAX request arrives with the product ID data.
PHP
add_action( 'wp_ajax_my_custom_action', 'my_custom_action_callback' );
add_action( 'wp_ajax_nopriv_my_custom_action', 'my_custom_action_callback' );
This code snippet registers two action hooks: wp_ajax_my_custom_action
and wp_ajax_nopriv_my_custom_action
. The first hook is for logged-in users, while the second is for non-logged-in users. Both hooks will trigger the same callback function, my_custom_action_callback
.
Inside the my_custom_action_callback
function, we’ll retrieve the product ID sent from the AJAX request and perform the necessary calculations to update the shopping cart total. Here’s an example:
PHP
function my_custom_action_callback() {
// Check for security nonce (explained later)
if ( ! wp_verify_nonce( $_POST['nonce'], 'my_custom_action_nonce' ) ) {
wp_send_json_error( array( 'message' => 'Invalid security nonce' ) );
die();
}
// Get the product ID from the request data
$product_id = intval( $_POST['product_id'] );
// Simulate fetching product price from database (replace with your logic)
$product_price = 10.00; // Assuming a price of £10.00
// Get the current cart total (replace with your logic)
$current_total = 0.00; // Assuming an initial cart total of £0.00
// Calculate the updated cart total
$updated_total = $current_total + $product_price;
// Prepare the response data
$response = array(
'data' => array(
'total' => $updated_total
)
);
// Send a successful JSON response with the updated total
wp_send_json_success( $response );
}
This function first verifies a security nonce (explained later) to prevent unauthorized requests. Then, it retrieves the product ID and simulates fetching the product price (replace this with your actual logic for retrieving product data). It then calculates the updated cart total based on the current total and the product price. Finally, it prepares a response containing the updated total in JSON format and sends it back to the client-side JavaScript code.
Back on the client-side, the success function of the AJAX request handles the server’s response. We can use the received data (updated total) to dynamically update the shopping cart total displayed on the page.
JavaScript
function updateCartTotal(newTotal) {
$("#cart-total h2").text("Cart Total: £" + newTotal.toFixed(2));
}
This function takes the new cart total as an argument and updates the content of the “Cart Total” heading with the formatted value.
This is a basic example showcasing the core functionalities of AJAX. It demonstrates how to send a request, process it on the server, and update the page dynamically without a full reload.
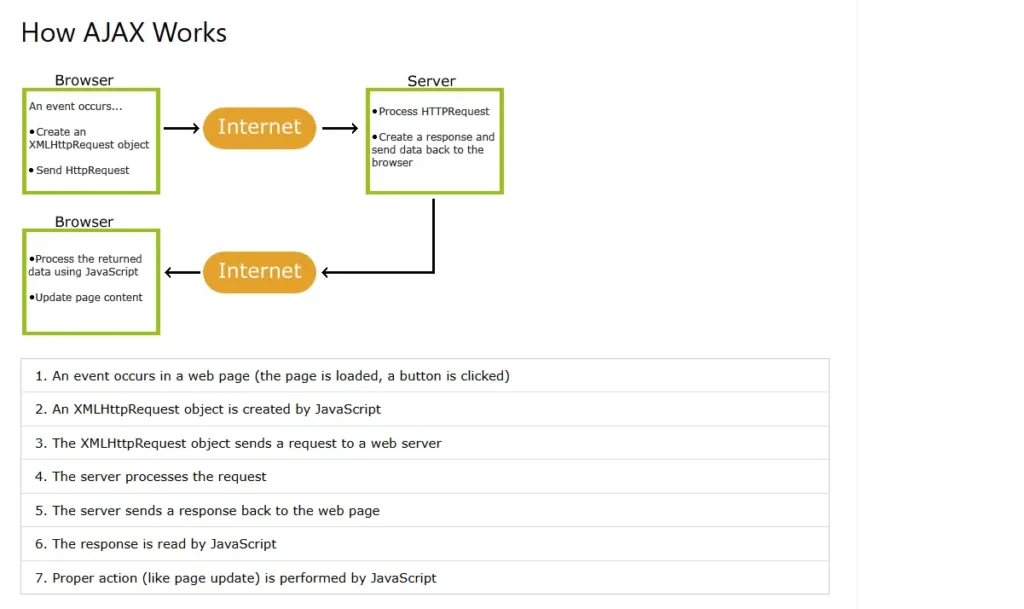
Advanced AJAX Techniques
Now that we have a grasp of the fundamentals, let’s explore some advanced techniques and considerations.
- Security: AJAX introduces security concerns as it involves communication beyond the initial page load. It’s crucial to implement security measures like nonces. Nonces are unique tokens generated on the server and sent along with the AJAX request. The server verifies the nonce before processing the request, mitigating the risk of unauthorized actions.
- Error Handling: Robust error handling is essential for a seamless user experience. The AJAX request and response functions should include mechanisms for handling potential errors (e.g., network issues, server errors). You can display user-friendly error messages or implement retry logic.
- Plugins: Several WordPress plugins simplify AJAX implementation. These plugins often provide helper functions and abstractions for common tasks, making development faster and easier.
- Advanced Use Cases: AJAX can be leveraged for various functionalities beyond basic content updates. For instance, you can implement live search functionality, infinite scrolling for content lists, and dynamic form validation with real-time feedback.
Conclusion
By incorporating AJAX into your WordPress development, you can significantly enhance user experience by enabling dynamic content updates and a more responsive feel. This blog post provided a foundational understanding of AJAX and its implementation, along with a practical example. As you delve deeper, explore advanced techniques, security considerations, and plugins to unlock the full potential of AJAX in your WordPress projects. Remember, continuous learning and exploration are key to mastering this powerful technology.
Interestingly, we recently used similar AJAX techniques to build a dynamic appointment scheduling system for a physiotherapy clinic AppliedMotion https://appliedmotion.com.au/podiatry-Wembley/, helping patients find a pain-free path to wellness. This is just one example of the many possibilities AJAX offers for creating engaging and user-friendly experiences.
Additional Resources:
- WordPress AJAX Documentation: https://developer.wordpress.org/plugins/hooks/actions/